In this tutorial you will create a game that tests the player’s reactions, initially a cross is shown on the Microbit and then every so often a tick will show on the Microbit. The player then has to press button “A” to score a point (if they press it when there isn’t a tick they lose two points).
Score
To keep the score you need to use a variable. A variable has a name and some information that it remembers, which you can change.
Click on “Variables” in the middle section and then on “Make a Variable”:
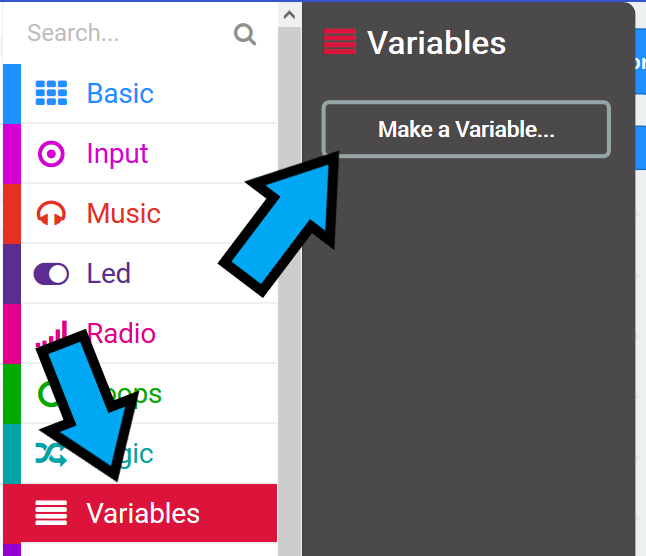
Type “Score” in the box that appears and then click “Ok”.
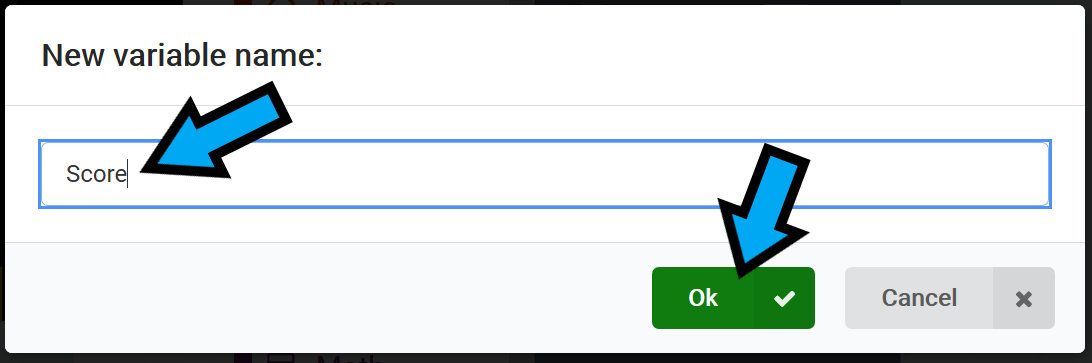
You’ve now created a variable called “Score”. Let’s make it so that pressing “A” increases the number remembered in “Score” and shows it to you.
Add the “on button A pressed” block (found by clicking on “Input” in the middle section) and put “show number 0” inside it (found by clicking on “Basic” in the middle section):
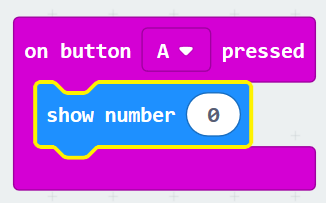
Now click on “Variables” in the middle section and click-and-drag “Score” so that it replaces the “0” in “show number 0”:
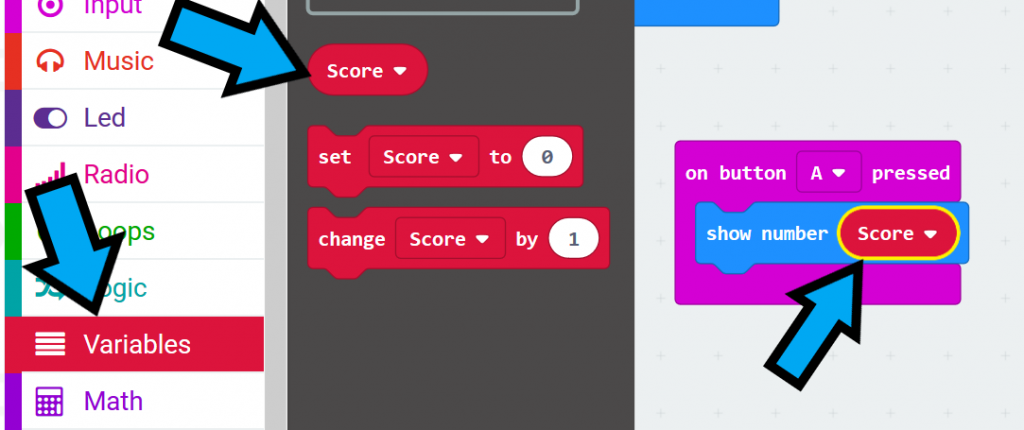
If you press “A” you just get 0 displayed every time as you never change “Score”. To fix this click on “Variables” in the middle section and click-and-drag the “change Score by 1” block into the block area inside “on button A pressed”, but put so that it clips just above “show number Score”:
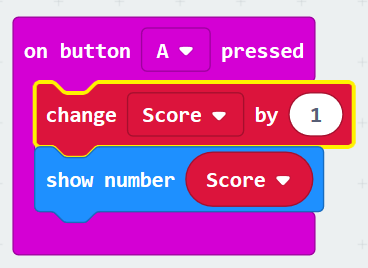
Now every time you press A you get shown an increasing number. You can make it increase faster by changing the “1” to a “3”. You can also make it decrease by changing the “1” to a “-1”.
To make sure “Score” starts at 0: Click on “Variables” in the middle section and click-and-drag “set Score to 0” so that it is inside “on start” (if you don’t have an “on start”, click on “Basic” in the middle section and click-and-drag “on start” into the block area from the blocks that appear). To make it set “Score” to 0, click on “item” in “set item to 0” and pick “Score” from the list that appears:
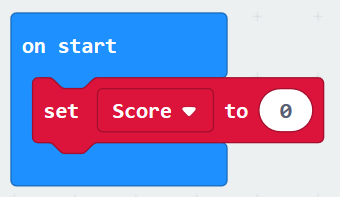
Showing the ticks and crosses
Click on “Loops” in the middle section and click-and-drag “while true” so that it clips to the bottom of “set Score to 0” inside “on start”:
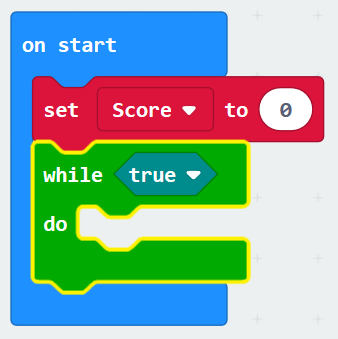
Whatever blocks are inside the “while true” block will happen again and again, until the block just after “while” isn’t true. At the moment that block is “true” so it will never stop.
Add a “show icon” block (click on “Basic” in the middle section) inside the “while true” block, and choose the cross icon:
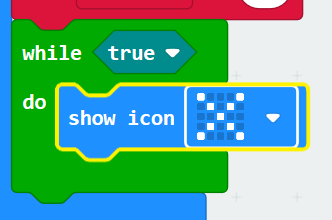
Click on “Basic” in the middle section and click-and-drag the “pause (ms) 100” block so that it clips just below “show icon”:
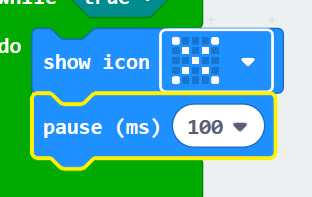
This will make the Microbit wait 100ms (or a tenth of a second) before doing the next block.
What is wanted is for the Microbit to wait a random amount of time between 1-5s and then show the tick. This is simple to do.
Click on “Math” in the middle section and click-and-drag “pick random 0 to 10” so that it replaces the “100” in the “pause (ms)” block.

Click on the “0” to and change it to “1000”, and change the “10” to “5000”.
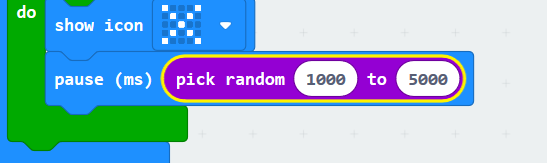
This “pause (ms) …” block now waits between 1s (1000ms) and 5s (5000ms).
Add a new “show icon” (in “Basic”) block and clip it just below the “pause” block, and set it to show a tick:
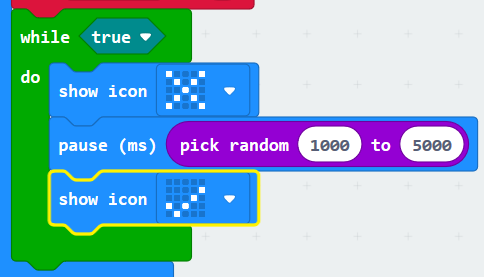
At the moment the tick disappears very quickly. Make it so that the tick shows for a short bit of time, by adding “pause (ms) 100” block just block the “show icon” block, and change the “100” to “200” (0.2s):
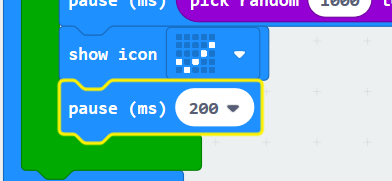
An accurate score
At the moment the score goes up whenever you hit “A” , even when a cross is showing. To fix that:
Add a new variable called “button been pressed” (click on “Variables” in the middle section and then on “Create Variable”).
Add a new “set button been pressed 0” block (found in “Variables” in the middle section) and clip it so it is just below “while true”, but above “show icon (cross)” in “on start”.
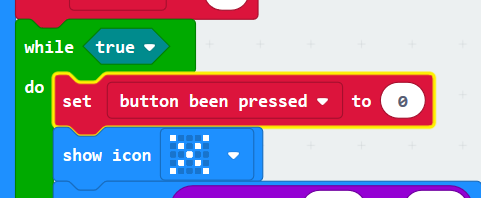
At the start there haven’t been any buttons pressed yet. Set “button been pressed” to false by clicking on “Logic” in the middle section and clicking-and-dragging “false” so that it replaces the “0” in “set button pressed to 0” block:
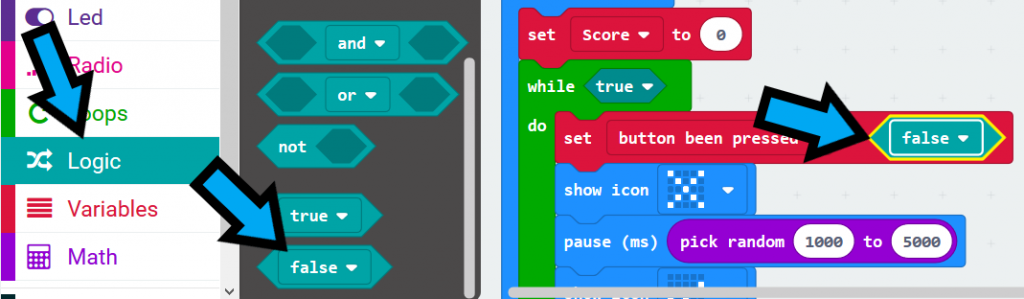
When a button is pressed you want “button been pressed” to be “true”. To do this add a new “set button been pressed to 0” block and put it at the very first block in the “on button A pressed” block:
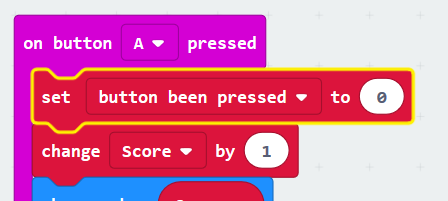
Click-and-drag “change Score by 1”, and all the blocks below it into the middle section to delete them, leaving just:
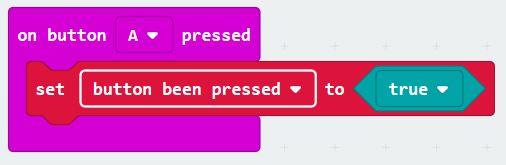
Add a new “if true then …” block and put it inside the “while true” block inside “on start” and clip it just below the first “pause (ms)” block. You might need to drag some blocks out of the “if true then …” block and put them just below it, as we want it to be empty:
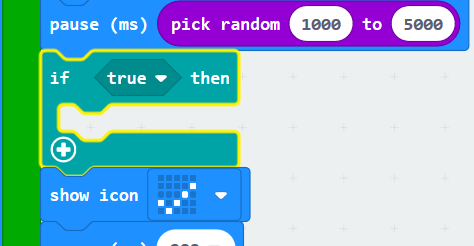
From “Variables” in the middle section click-and-drag “button been pressed” and use it to replace the “true” in the block you just added.
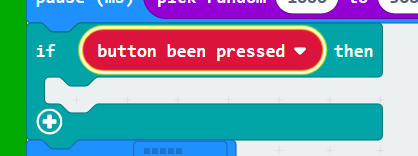
If a button has been pressed while the cross was showing the score should be decreased by 2 points. To do this add a new “change button been pressed by 1” block and put it inside the if block:
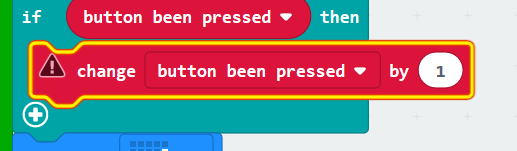
Now click on “button been pressed” and a list will appear, click on “Score” to use the “Score” variable instead:
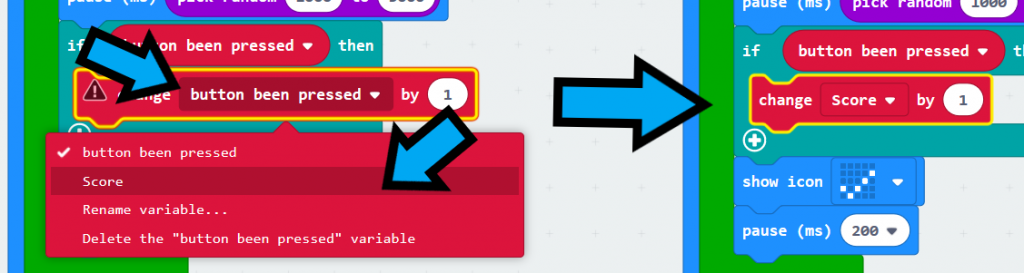
Click on “1” and change it to “-2” to decrease the score by 2.
Add a new “set button been pressed to 0” block and put it just below the if block, and replace the “0” with “false” (found in “Logic” in the middle section).
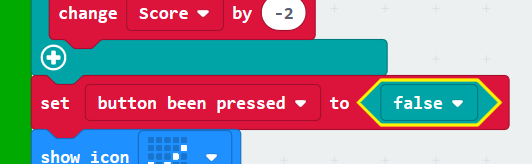
Click on “Logic” again and click-and-drag another “if true then …” block and clip it just below the “pause (ms) 200” block:
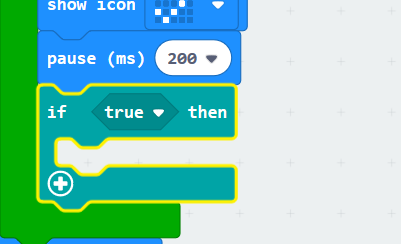
Just like in the previous “if …” block, replace the “true” with “button been pressed”, and setup a “change Score by 1” block inside it, but this time don’t change the “1”.
Ending the game
At the moment the game lasts forever. It should end at some point. Maybe after 30 seconds?
To figure out when enough time has passed you can record how long the game as been running by using a variable. It will remember how long you have used “sleep (ms)”) for. This isn’t 100% accurate, but it is pretty good.
Create a new variable called “time” (click on “Variables in the middle section and then on “Create Variable”), and add a “set time to 0” block just below “set Score to 0” in “on start”:
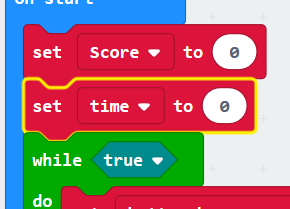
Just one more variable is needed, create it and call it “random sleep”.
Just before the first “sleep (ms)” inside “on start” add a “set random sleep to 0” block:
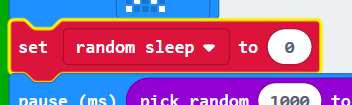
Now click-and-drag the “pick random 1000 to 5000” out of the first “sleep (ms)” block just below the block you added and use it to replace the “0” in the block you just added:

Click on “Variables” in the middle section and click-and-drag “random sleep” and use it to replace the “0” in the first “sleep (ms)”:
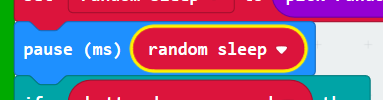
If you run the blocks as they are now you won’t notice any difference, as it still sleeps for a random amount of time. It’s just remembered by a variable now.
Just below the “set random sleep to …” block add a “change random sleep by 0” block (found in “Variables” in the middle section) and change “random sleep” to “time” and replace the “0” with “random sleep”. This adds the random sleep time to the variable that remembers how long the game has been running for:
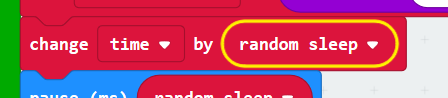
Now add another “change random sleep by 0” block and clip it just above the next “sleep (ms)” block and change “random sleep” to “time” and change the “0” to “200”:
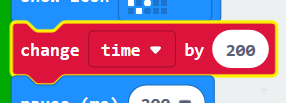
To make the loop stop when “time” reaches 30s you need to change the “true” in the “while true” block to something else.
Click on “Logic” in the middle section and click-and-drag “0 < 0” and make it replace “true” in the “while true” block:
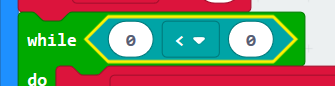
Replace the first “0” by “time” (found in “Variables” in the middle section) and change the second “0” to “30000” – “time” is counted in milliseconds:
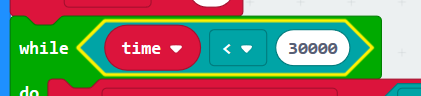
If you play the game you will find it stops after 30,000ms (or 30s).
Showing the score
To do this click on “Basic” in the middle section and then click-and-drag “show number 0” and clip it just below the “while” block in “on start” – so that it is the last block there.
Now replace the “0” with “Score” (found in “Variables” in the middle section):
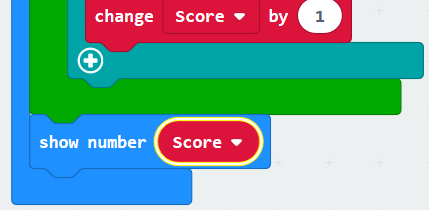
That’s it
You now have a fully functioning game. You could make it last longer or less time, but it’s good enough as it is.
The completed game can be found here.
The next tutorial creates a game of ping-pong.