Every website that you see is written using HTML (Hyper-Text Markup Language).
HTML is a language that defines the content of a webpage. It is usually combined with CSS (Cascading Style Sheets) which specifies how the page should look, including colours, whether text is bold or borders.
An element of a HTML page can be images, headings, paragraphs or plain text.
This tutorial will use HTML5 compliant HTML, this may look slightly different to the HTML used tutorials elsewhere, but works just about everywhere, and mostly works in ancient web browsers.
The core HTML page
<!doctype html> <html> <head> <meta charset="UTF-8"> <title> First webpage </title> </head> <body> Hello World! </body> </html>
This HTML forms the basis of every html page with the emphasised text (‘First webpage’ and ‘Hello World!’) being swapped out for something else depending on the website.
Type or copy the HTML code into a plain text editor (see here) and save the file as “first.html”. If you have the option, choose the encoding to be UTF-8.
Using your web browser open the saved file, using File->Open, Ctrl+O or Cmd+O to locate your file.
The HTML entered told the web browser that the page’s title is ‘First Webpage’ and that its page content is ‘Hello World!’, which is exactly what appears.
You should see something similar to the below (excluding the arrows):
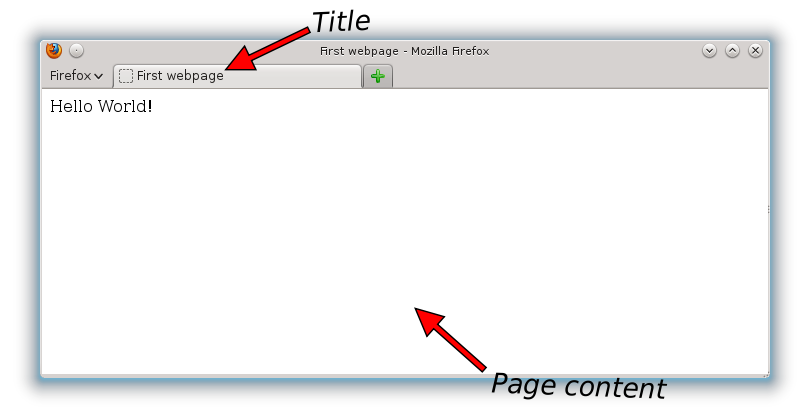
HTML is made up of things called tags with each tag looking like <
[tag name]>
. You can see that this makes <!doctype html>
, <html>
, <head>
, <meta charset="UTF-8">
, <title>
and <body>
tags. These particular tags must exist on every HTML page.
The html
tag indicates the start of the page, head
contains stuff that normally displayed, and the title
which doesn’t appear in the page, but instead in the title bar. Finally body
contains all the stuff that gets shown such as text, videos and images.
The <!doctype html>
tag tells the web browser to interpret the file as correct HTML and <meta charset="UTF-8">
tells the web browser what file encoding (computer format used to represent a character) the webpage is stored in, in this case it UTF-8, the recommended encoding.
Most tags have another similar tag to follow them of the form </
[tag name]>
. This is the closing tag, and is a requirement. For </html>
it indicates the end of the page, for </head>
it is the end of the stuff do do with head
, </title>
is to mark the end of the title text and </body>
indicates the end of the stuff that is shown.
The first tag that starts something like <body>
is called the opening tag.
Some tags do not have a closing tag like <br>
(mentioned later) they do something unusual.
Altering the page
The title ‘First Webpage’ isn’t a great title. It is easily changed though. Just change the text inside the title
tags. To make the title “Website 1” that would give:
<title>Website 1</title>
The text “Hello World!” is the classic first program for new programmers. That can be swapped out for something else without any trouble:
<body> This is my <em>new</em> website </body>
You will notice a new tag <em>
that emphasises the text by making the text italic. In this case the word “new” has been emphasised. Tags only affect the stuff between their opening (<em>
) and closing (</em>
) tags. If you forget to close the tag it will affect the rest of the page.
Another tag is <strong>
which indicates that text is important, by making the text bold:
<body> This is <strong>my</strong> <em>new</em> website </body>
Putting all the modifications together gives:
<!doctype html> <html> <head> <meta charset="UTF-8"> <title> Website 1 </title> </head> <body> This is <strong>my</strong> <em>new</em> website <body> </html>
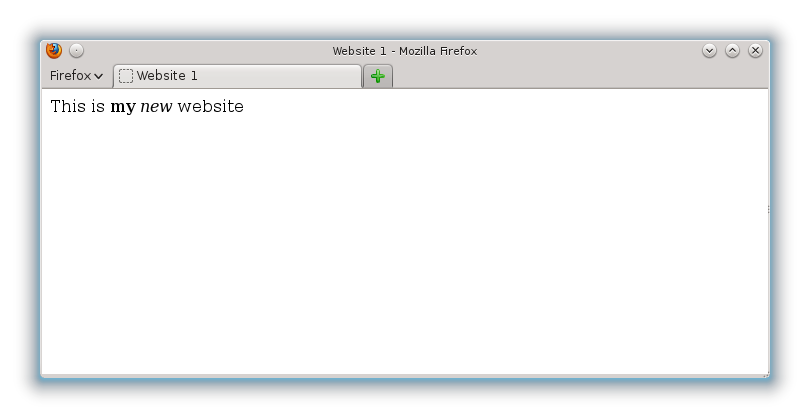
This results in:
Connecting web pages (using hyperlinks)
You need to make
Three pages, called home.html, page1.html and page2.html, and all in the same directory/folder.
Put the basic HTML tags in them (remember the core HTML tags and <!doctype html>
, see the first earlier) and put some interesting text to be displayed.
The home.html file that this tutorial uses is shown below:
home.html
<!doctype html> <html> <head> <meta charset="UTF-8"> <title>Home</title> </head> <body> This is the homepage </body> </html>
It will be setup that home.html will link (connect) to page1.html and page2.html, page1.html will link to page2.html and page2.html will link to home.html.
Creating the connections
In HTML links (connections) from one page to another are done using a hyperlink tag <a>
, that makes clickable text or images that will make the web browser open another page when they’re clicked
This tag has a required attribute, which is something that you set on the tag to make it do something the web browser can’t guess, like setting the web page to link to.
The completed tag to like to page1.html called ‘Link to page 1’ would be:
<a href="page1.html">Link to page 1</a>
The bold text page1.html is the linked page which is what the attribute href
is set to. Its value can be changed to say page2.html to link to there instead. Naturally the text ‘Link to page 1‘ can be changed.
For another website the full website URL (web address), which is prefixed with http:// or https:// is the attribute’s value. This gives href="https://www.invincitech.com"
to link to this website and not href="www.invincitech.com"
, which won’t work. Links to other parts of the website do not need to specify the full web address, like the link for page2.html shown below:
<a href="page2.html">Link to the 1st page - excluding the homepage</a>
The text for the link should be changed to say where it actually goes:
<a href="page2.html">Link to the 2nd page - excluding the homepage</a>
Try making a link to home.html yourself, and then check below to see if you did it correctly:
home.html
<!doctype html> <html> <head> <meta charset="UTF-8"> <title>Home</title> </head> <body> This is the homepage. <br> Here is a link to <a href="page1.html">Page 1</a> <br> And here is link to <a href="page2.html">the <em>second</em> page</a> </body> </html>
This will give:
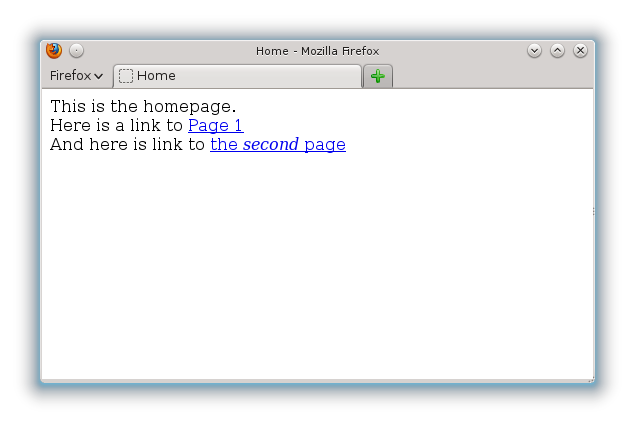
A new tag <br>
In home.html, for example purposes the tag, <br>
was used, which instructs the web browser to insert a new line at its position. It is needed if a newline is required as newlines in a HTML file are ignored. It isn’t needed unless a line is specifically wanted at its position; the browser will automatically insert newlines to stop text going off the screen.
A better method for paragraphs is shown in the next part.
All your HTML code can be placed on the same line or on different lines, it makes no difference to how it is displayed. Also note that the tag <br>
does not have an equivalent </br>
as it only indicates a new line and doesn’t change the behaviour of other page elements.
Tag nesting
Tags can be nested inside each other, in home.html <strong>
was inside <a>
which was inside <body>
which was inside <html>
. Each tag should be closed in reverse order of opening, as is done in home.html.
The following code is invalid because it does not follow that rule (it will function as you expect it to, but will fail the HTML validator, see http://validator.w3.org/). Following the rule eases comprehension of HTML code, and the web browser’s job as it does not have guess as to your indent, although it usually does so correctly:
<strong><em>This is</strong> partially important text</em>next part
That should have been:
<em><strong>This is</strong> partially important text</em>
Linking to a page in another directory
The directory (folder) where the page is located must be named. For a subdirectory it is named in the form, “subdir/page.html” and can be several directories deep, “subdir/anothersubdir/andanother/thepage.html”.
If the page is in a subdirectory and it wishes to link to a page in its parent directory it can do so using ../ to refer to the parent directory (../../ will refer to the parent of the parent directory, ../../../ goes up three levels). You can set href to ../parentpage.html to link to parentpage.html in the parent directory (href=”../parentpage.html”). Subdirectories of the parent directory can be linked to (../subdir). If the page (text.html) is in the subdir2 directory the page in the subdir directory, both of which are subdirectories of ‘parent’, can be referred to using ../subdir/test.html.
<a href="subdir/subdir2/pg.html">Link to page two subdirs deep</a> <a href="../subdir/pg2.html">Link to a page in a subdir of the parentdir</a>
The other pages…
Are left as an exercise.